· 3 min read
GraphQL Mutation with API Key Example
A simple example that demonstrates how to check for API Key in the case of graphQL
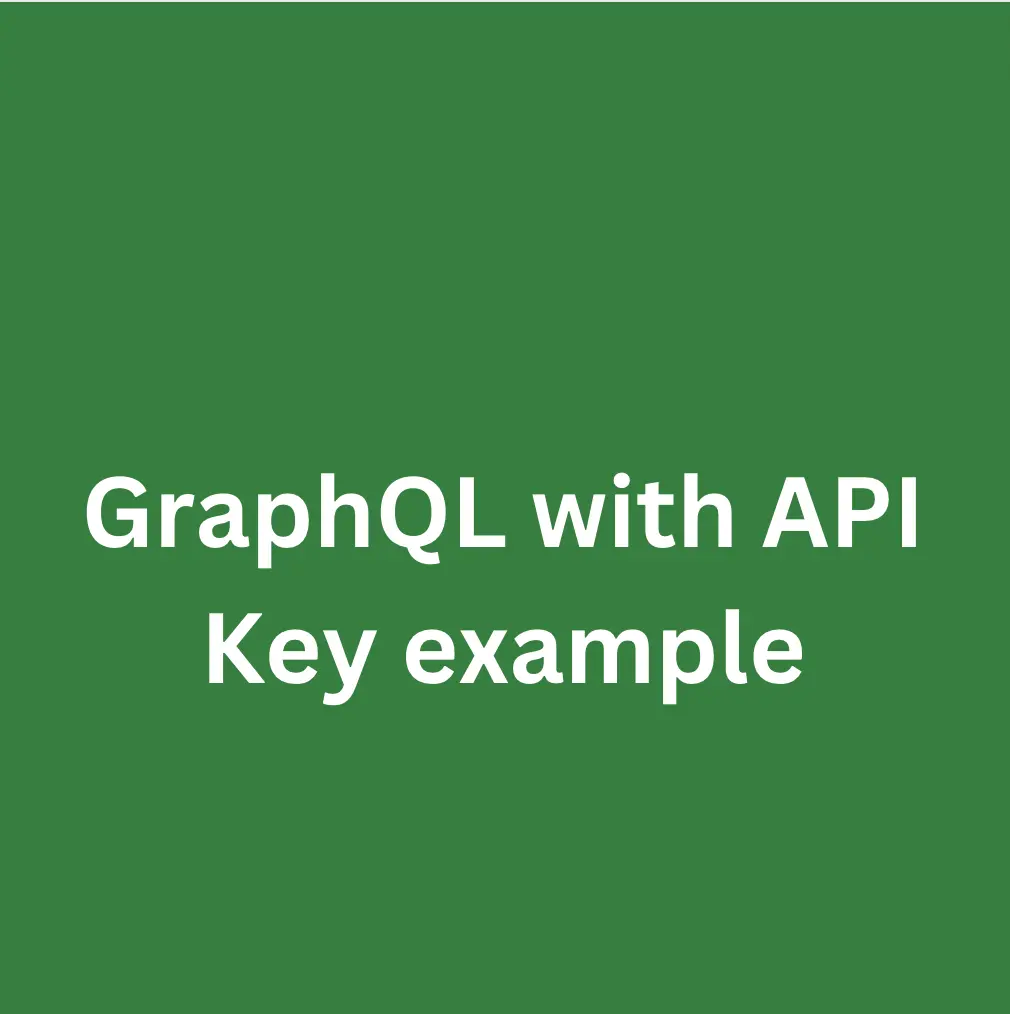
Why GraphQL over the popular REST
GraphQL and REST are both widely used approaches for building APIs, but they have some fundamental differences. Here are some advantages of GraphQL over REST:
- Efficient data fetching: With REST, you often have to make multiple requests to different endpoints to fetch all the data you need. In contrast, GraphQL allows you to request only the data you need in a single request. This can significantly reduce network overhead and improve performance.
- Strongly-typed schema: GraphQL uses a strongly-typed schema to define the types of data that can be queried and the relationships between them. This makes it easier to understand and use the API, and can help prevent errors.
- Client-driven queries: With REST, the server determines the structure of the data that is returned in response to a request. In contrast, GraphQL allows the client to define the structure of the data it needs, which can make it easier to build client applications.
- Versioning: With REST, versioning can be complex and requires creating separate endpoints for each version. With GraphQL, the schema can be versioned and evolved over time without breaking existing clients.
- Developer experience: GraphQL has a number of developer-friendly features, such as the ability to introspect the schema and generate documentation and type-safe client code. This can help improve developer productivity and reduce errors.
Overall, GraphQL can provide a more flexible, efficient, and developer-friendly approach to building APIs compared to REST. However, the choice between GraphQL and REST ultimately depends on the specific needs of your application and team.
Example
Here’s an example of a GraphQL mutation resolver that requires an API key to be passed in as an argument. This example assumes that you’re using Node.js and the Apollo Server library.
const { AuthenticationError } = require('apollo-server');
// Define the GraphQL mutation type
const typeDefs =
// Define the resolver for the mutation
const resolvers = {
Mutation: {
updateProfile: async (parent, args, context) => {
// Check if the API key is included in the request headers
const apiKey = context.req.headers['api-key'];
if (!apiKey) {
throw new AuthenticationError('API key missing');
}
// Verify that the API key is valid
const isValidApiKey = await verifyApiKey(apiKey);
if (!isValidApiKey) {
throw new AuthenticationError('Invalid API key');
}
// Update the user's profile
const { firstName, lastName } = args;
const updatedProfile = await updateProfile(context.userId, firstName, lastName);
return updatedProfile;
},
},
};
// Verify that the API key is valid
async function verifyApiKey(apiKey) {
// Query your database or API service to validate the API key
// Return true if the API key is valid, false otherwise
// You can use a library like
// Update the user's profile in the database
async function updateProfile(userId, firstName, lastName) {
// Update the user's profile in the database
// Return the updated profile object
}
// Create the Apollo Server instance
const server = new ApolloServer({
typeDefs,
resolvers,
context: ({ req }) => {
// Extract the user ID from the request headers, assuming you have previously authenticated the user
const userId = req.headers['user-id'];
// Return the context object with the user ID and request object
return { userId, req };
},
});
// Start the Apollo Server
server.listen().then(({ url }) => {
console.log((`🚀 Server ready at ${url}`);
});
In this example, the updateProfile mutation requires an API key to be included in the request headers. If the API key is missing or invalid, an AuthenticationError is thrown. If the API key is valid, the mutation updates the user’s profile in the database and returns the updated profile object. The verifyApiKey function is where you would typically query your database or API service to validate the API key.