· 4 min read
Incremental Static Regeneration(ISR)
ISR operates by creating a "stale-while-revalidate" mechanism. When a user requests a page that needs to be regenerated, Vercel will serve the previously generated version ("stale") while initiating a background process to generate an updated version ("revalidate").
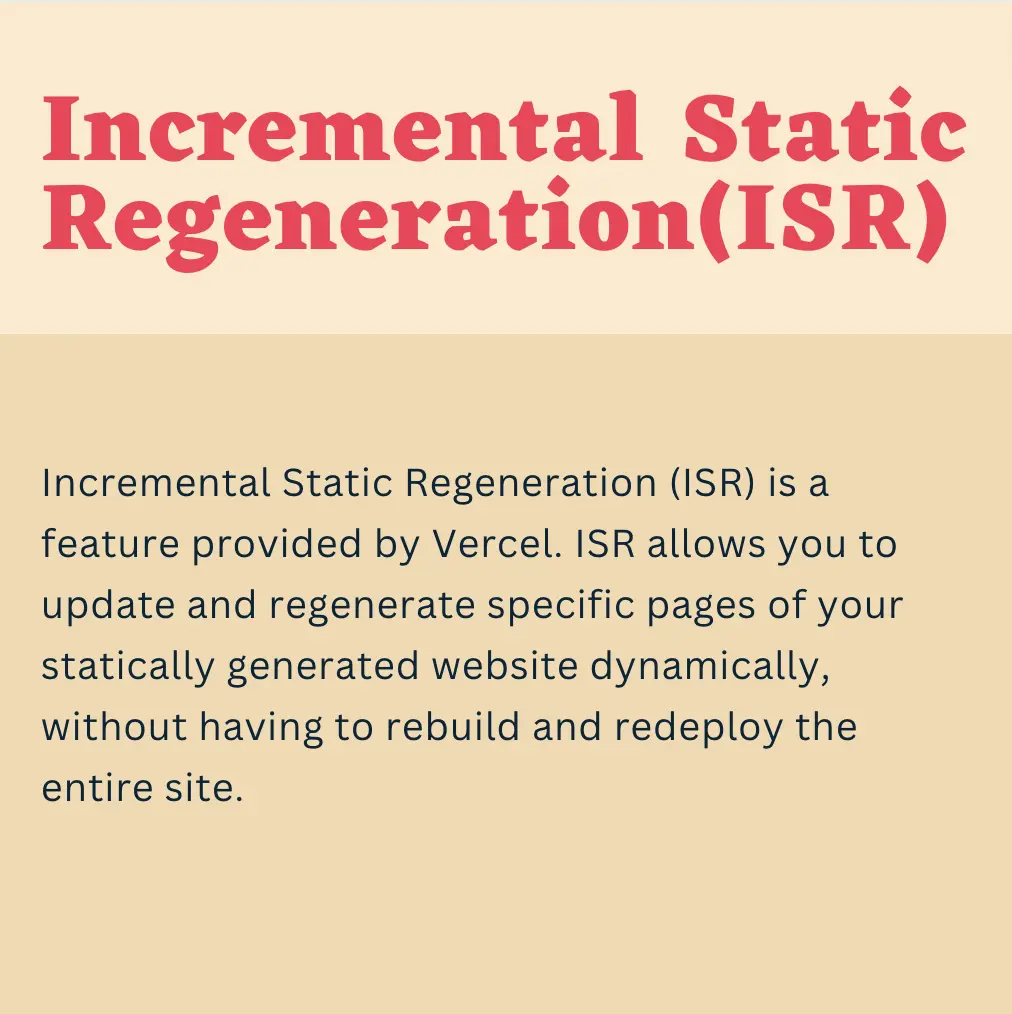
Incremental Static Regeneration (ISR) is a feature provided by Vercel, a cloud platform for deploying web applications. ISR allows you to update and regenerate specific pages of your statically generated website dynamically, without having to rebuild and redeploy the entire site.
In a statically generated website, the pages are pre-rendered during the build process and served as static files. This approach offers fast and efficient performance as the content is served directly from the edge locations. However, it can be challenging to update specific pages that require dynamic data, such as a blog with comments or a news website with frequently changing articles.
With ISR, Vercel introduces a solution to this problem. When a user requests a page that has not been generated or has expired (based on a defined time interval), Vercel will dynamically generate the page on-demand and cache it for subsequent requests. This ensures that the static pages remain fast and cacheable while allowing for dynamic updates.
ISR operates by creating a “stale-while-revalidate” mechanism. When a user requests a page that needs to be regenerated, Vercel will serve the previously generated version (“stale”) while initiating a background process to generate an updated version (“revalidate”). Once the new version is ready, subsequent requests will receive the regenerated page, ensuring the latest data is displayed.
The benefit of ISR is that it strikes a balance between static site performance and dynamic content updates. It allows you to keep most of your site as static files for optimal speed, while still enabling dynamic parts to be updated in real-time or with a predefined interval.
By leveraging ISR in Vercel, you can provide users with fast-loading static pages while ensuring that certain parts of your website can be updated dynamically without the need for full rebuilds and deployments. This makes it easier to maintain and deliver content that requires frequent updates while maximizing performance and scalability.
Sample ISR code blog post and comments
In the code that handles comment submissions, you’ll need to configure ISR to regenerate the comments section of the blog post when new comments are submitted. Here’s an example code snippet using the Next.js framework and Vercel’s ISR:
import { getCommentsForPost } from 'your-comment-service'; // Replace with your own comment service
export async function getStaticProps({ params }) {
const { postId } = params;
// Fetch the blog post content
const post = await fetchPostContent(postId);
// Fetch the comments for the post
const comments = await getCommentsForPost(postId);
return {
props: {
post,
comments,
},
revalidate: 60, // Regenerate the page every 60 seconds
};
}
export async function getStaticPaths() {
// Fetch the list of blog post IDs
const postIds = await fetchPostIds();
// Generate the paths for the blog post pages
const paths = postIds.map((postId) => ({
params: { postId },
}));
return {
paths,
fallback: true, // Enable fallback for paths not generated at build time
};
}
export default function BlogPost({ post, comments }) {
// Render the blog post and comments
return (
<div>
<h1>{post.title}</h1>
<div>{post.content}</div>
<h2>Comments</h2>
<ul>
{comments.map((comment) => (
<li key={comment.id}>{comment.text}</li>
))}
</ul>
{/* Add a comment submission form here */}
</div>
);
}
In the code snippet above, getStaticProps
is responsible for fetching the blog post content and comments. It uses the revalidate
option to specify the interval (in seconds) at which the page should be regenerated.
The getStaticPaths
function generates the paths for the blog post pages based on the available post IDs. The fallback: true
option enables fallback behavior, allowing pages that haven’t been generated at build time to be generated on-demand.
The BlogPost
component renders the blog post content and comments. It can also include a comment submission form.
With this setup, when a user requests a blog post page, Vercel will serve the static version of the page initially. If a new comment is submitted, Vercel will automatically regenerate the page in the background based on the specified revalidation interval. Once the new version is ready, subsequent users will receive the updated page with the latest comments.