· tutorials · 4 min read
Authentication with JWT vs API Key
Express App Authentication: JSON Web Tokens (JWTs) & API keys for secure requests. Verify authenticity via tokens/keys. Learn more.
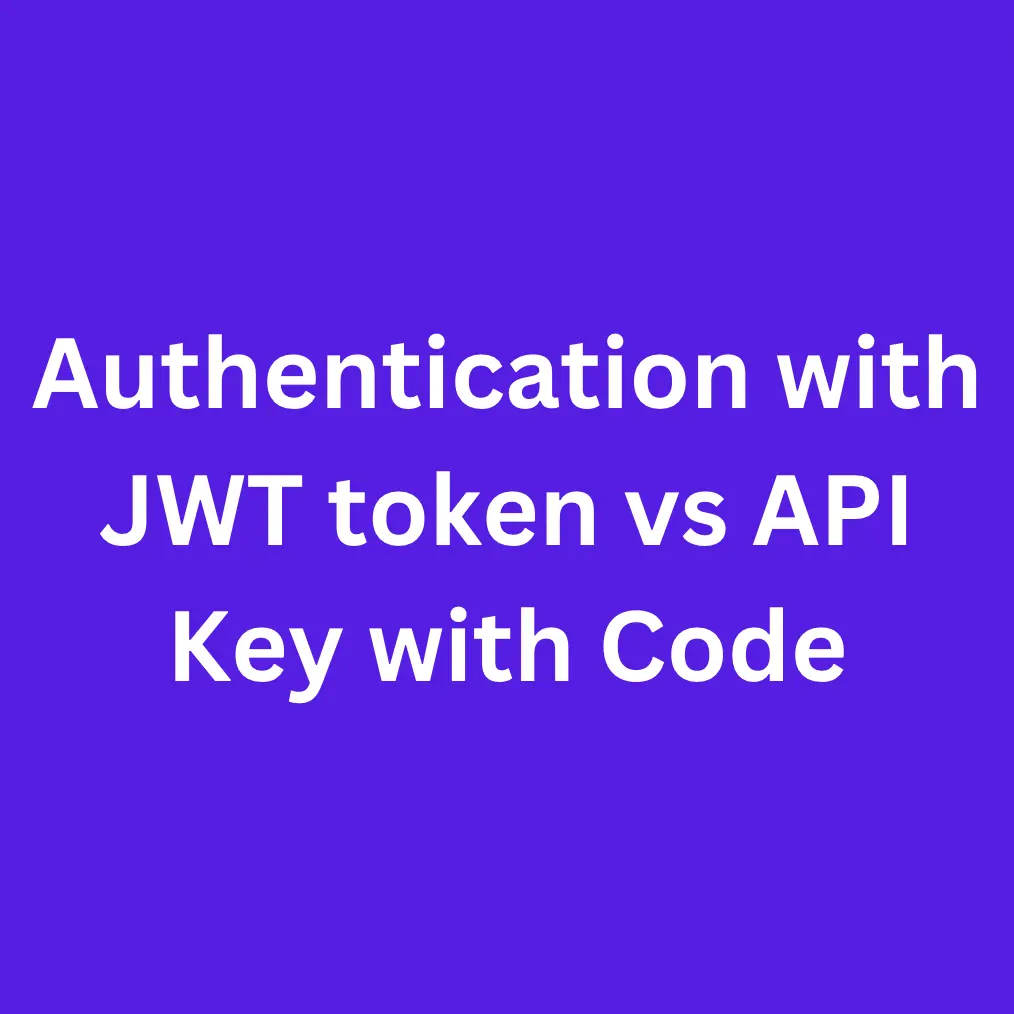
In an Express application, authentication can be implemented in multiple ways, including using JSON Web Tokens (JWTs) and API keys. Both methods involve sending a token or key with each API request to verify the authenticity of the request.
JSON Web Tokens (JWTs) are a popular method for implementing authentication in modern web applications. They are self-contained tokens that contain user information and are signed using a secret key. When a user logs in, a JWT is generated on the server and sent back to the client, which can then use the token to make authenticated API requests. Here’s an example of how to implement JWT authentication in an Express application:
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
// Secret key for signing JWTs
const secretKey = 'mySecretKey';
// Login endpoint
app.post('/login', (req, res) => {
// Get user credentials from request body
const { username, password } = req.body;
// Check if credentials are valid
if (username === 'myUsername' && password === 'myPassword') {
// Generate JWT and send it back to the client
const token = jwt.sign({ username }, secretKey);
res.json({ token });
} else {
res.status(401).send('Invalid credentials');
}
});
// Protected endpoint that requires JWT authentication
app.get('/protected', (req, res) => {
// Get JWT from authorization header
const authHeader = req.headers.authorization;
if (!authHeader) {
return res.status(401).send('Authorization header missing');
}
const token = authHeader.split(' ')[1];
// Verify JWT and extract username
try {
const decoded = jwt.verify(token, secretKey);
const { username } = decoded;
// Do something with the authenticated user
res.send(Hello, ${username});
} catch (err) {
res.status(401).send('Invalid token');
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In the above example, the /login
endpoint checks the user credentials and generates a JWT using the jwt.sign()
method from the jsonwebtoken
library. The generated JWT is then sent back to the client in the response body.
The /protected
endpoint is protected and requires JWT authentication. When a client makes a request to this endpoint, the server first checks for the presence of an authorization header in the request. If the header is missing, the server responds with a 401 Unauthorized
status code. If the header is present, the server extracts the JWT from the header and verifies it using the jwt.verify()
method. If the JWT is valid, the server extracts the username from the JWT payload and sends a response back to the client.
API keys are another method for implementing authentication in web applications. API keys are essentially tokens that are generated by the server and sent to the client. The client includes the API key with each request to the server to authenticate the request. Here’s an example of how to implement API key authentication in an Express application:
const express = require('express');
const app = express();
// API key middleware
const apiKeyMiddleware = (req, res, next) => {
// Get API key from request header or query parameter
const apiKey = req.headers['x-api-key'] || req.query['apiKey'];
if (!apiKey) {
return res.status(401).send('API key missing');
}
// Check if API key is valid
if (apiKey === 'myApiKey') {
// API key is valid, continue with request
next();
Folder structure for the API-key middleware in Express.JS
Here’s an example folder structure for an Express application that uses the API key middleware:
├── node_modules/
├── app.js
├── controllers/
│ └── protectedController.js
├── middleware/
│ └── apiKeyMiddleware.js
└── package.json
In this folder structure, app.js
is the main entry point for the Express application. It’s where the application is initialized and where the routes and middleware are registered.
The controllers
folder contains the controllers for each route in the application. In this example, there’s a protectedController.js
file that handles requests to the protected endpoint.
The middleware
folder contains the middleware functions that are used in the application. In this example, there’s a apiKeyMiddleware.js
file that checks for the presence of an API key in the request headers or query parameters.
Here’s an example of how the app.js
file might look like with the apiKeyMiddleware
:
const express = require('express');
const apiKeyMiddleware = require('./middleware/apiKeyMiddleware');
const protectedController = require('./controllers/protectedController');
const app = express();
// Register API key middleware
app.use(apiKeyMiddleware);
// Protected endpoint that requires API key authentication
app.get('/protected', protectedController);
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, the apiKeyMiddleware
is registered using the app.use()
method. This ensures that the middleware is applied to every request that comes through the application.
The protectedController
handles requests to the protected endpoint. The apiKeyMiddleware
checks for the presence of an API key in the request and ensures that the key is valid before allowing the request to continue to the protectedController
.
With this folder structure and code, you can implement API key authentication in your Express application.