· tutorials · 10 min read
Top 10 state management for React Native in 2024
State management libraries like redux, mobx, zustland provide a way to manage the state of your application in a more centralized and organized way.
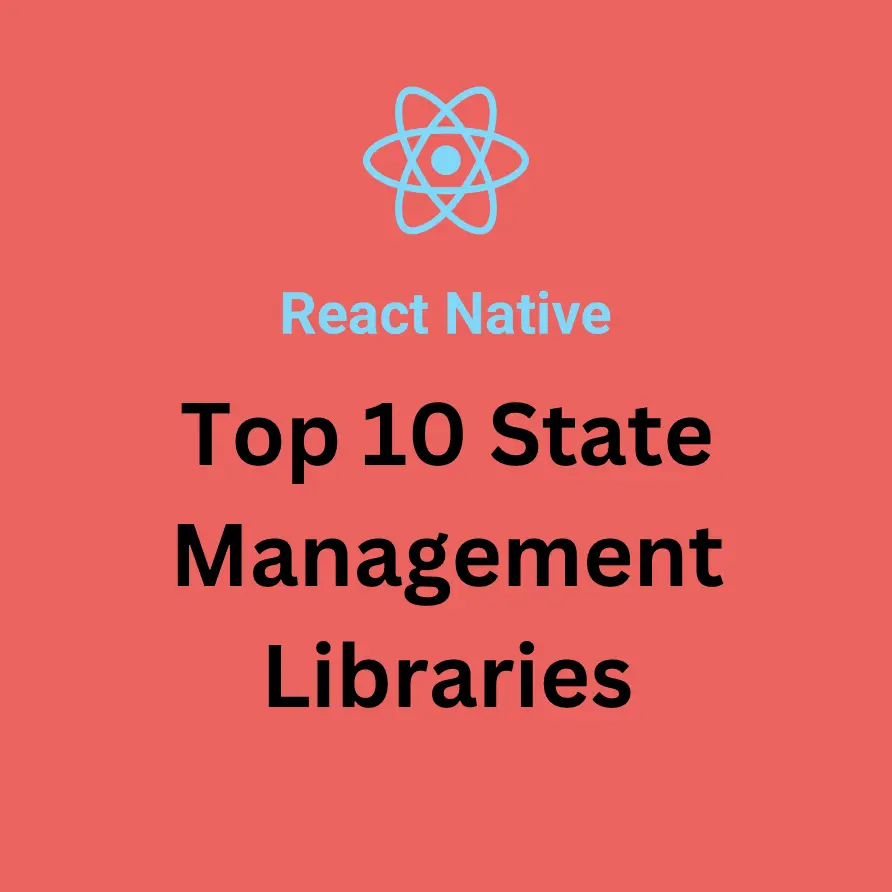
React state management libraries solve the problem of managing the state of a React application. State is the data that changes over time in your application. For example, the state of a todo list application might include the list of todos, the current todo that is being edited, and the user’s preferences.
Without a state management library, you would have to manage the state of your application manually. This would involve storing the state in individual components, and then updating the state whenever it changed. This can be a tedious and error-prone process, especially as your application grows in size and complexity.
State management libraries provide a way to manage the state of your application in a more centralized and organized way. This can make it easier to understand and maintain your application’s state, and it can also make it easier to debug your application.
Here are some of the problems that React state management libraries solve:
-
State management can be complex and error-prone: Managing state manually can be a complex and error-prone process. This is especially true as your application grows in size and complexity.
-
State management can be difficult to debug: When state is managed manually, it can be difficult to track down the source of problems when something goes wrong.
-
State management can impact performance: If state is managed manually, it can impact the performance of your application. This is because React components will re-render whenever the state changes.
State management libraries can help to solve these problems by providing a centralized and organized way to manage state. This can make it easier to understand and maintain your application’s state, and it can also make it easier to debug your application. Additionally, state management libraries can help to improve the performance of your application by reducing the number of re-renders that occur.
Here are the top 10 React Native state management libraries:
1. Redux
Redux is a popular state management library that is known for its simplicity and flexibility. It is based on the Flux architecture, which makes it easy to reason about and debug your state.
Pros:
- Simplicity and flexibility
- Well-documented and supported
- Large community of users and developers
- Extensive ecosystem of third-party libraries
Cons:
- Can be complex to learn and use
- Not as performant as some other libraries
- Can be difficult to debug
2. Mobx
MobX is another popular state management library that is known for its performance and simplicity. It is based on the observable pattern, which makes it easy to subscribe to changes in your state management library
Pros:
- Performance
- Simplicity
- Easy to learn and use
- Observable pattern makes it easy to subscribe to changes in state
Cons:
- Not as well-documented as Redux
- Smaller community of users and developers
- Ecosystem of third-party libraries is not as extensive as Redux
3. Zustland
Zustand is a lightweight state management library that is based on the Context API. It is easy to learn and use, and it is well-suited for small to medium-sized applications.
Pros:
- Lightweight and performant
- Easy to learn and use
- Well-documented and supported
- Large community of users and developers
Cons:
- Not as powerful as some other libraries
- Can be difficult to debug large applications
4. Jotai
Jotai is a new state management library that is gaining popularity. It is based on the observable pattern, and it is very lightweight and performant.
Pros:
- Lightweight and performant
- Easy to learn and use
- Observable pattern makes it easy to subscribe to changes in state
- New and actively developed, so it is likely to improve over time
Cons:
- Not as well-documented as some other libraries
- Smaller community of users and developers
- Ecosystem of third-party libraries is not as extensive as other libraries
5. Recoil
****
Recoil is a state management library that is built on top of React’s Context API. It is very lightweight and performant, and it is easy to learn and use.
Pros:
- Lightweight and performant
- Easy to learn and use
- Built on top of React’s Context API, so it is easy to integrate with existing code
- Actively developed and maintained by the React team
Cons:
- Not as well-documented as some other libraries
- Smaller community of users and developers
- Ecosystem of third-party libraries is not as extensive as other libraries
6. Akita
Akita is a state management library that is based on the Redux pattern. It is well-suited for large applications, and it provides a number of features that are not available in other libraries, such as code-splitting and hot reloading react native state management library
Pros:
- Well-suited for large applications
- Provides a number of features that are not available in other libraries, such as code-splitting and hot reloading
- Actively developed and maintained by the Akita team
Cons:
- Can be complex to learn and use
- Not as well-documented as some other libraries
- Smaller community of users and developers
7. Hookstate
Hookstate is a state management library that is based on React hooks. It is very easy to learn and use, and it is well-suited for small to medium-sized applications.
Pros:
- Very easy to learn and use
- Based on React hooks, so it is easy to integrate with existing code
- Actively developed and maintained by the Hookstate team
Cons:
- Not as powerful as some other libraries
- Can be difficult to debug large applications
8. Easy-peasy
Easy-peasy is a state management library that is based on the Context API. It is very easy to learn and use, and it is well-suited for small to medium-sized applications.
Pros:
- Very easy to learn and use
- Based on the Context API, so it is easy to integrate with existing code
- Actively developed and maintained by the Easy-peasy team
Cons:
- Not as powerful as some other libraries
- Can be difficult to debug large applications
9. Valtio
Valtio is a state management library that is based on the Context API. It is very lightweight and performant, and it is easy to learn and use.
Pros:
- Lightweight and performant
- Easy to learn and use
- Based on the Context API, so it is easy to integrate with existing code
- Actively developed and maintained by the Valtio team
Cons:
- Not as well-documented as some other libraries
- Smaller community of users and developers
- Ecosystem of third-party libraries is not as extensive as other libraries
10. React Context API
React Context API is a built-in state management library that is provided by React. It is very easy to learn and use, but it is not as powerful as other libraries.
Pros:
- Very easy to learn and use
- Built-in to React, so it is easy to integrate with existing code
Cons:
- Not as powerful as some other libraries
- Can be difficult to debug large applications
Principles of state management in react native state management libraries
Here are some of the principles of state management in React Native state management libraries:
Immutability
The state of your application should be immutable. This means that once you have set the state, you should not be able to change it directly. Instead, you should create a new state object with the changes you want to make. This makes it easier to reason about your state and to track down bugs.
Encapsulation
The state of your application should be encapsulated. This means that the state should be hidden from the components that do not need to know about it. This makes it easier to maintain your application and to prevent components from depending on each other in unexpected ways.
Decoupling
The state of your application should be decoupled from the UI. This means that the state should not be directly connected to the UI. Instead, the UI should be connected to the state through a series of functions called selectors. This makes it easier to test your UI and to make changes to the state without affecting the UI.
Single source of truth
There should be a single source of truth for the state of your application. This means that all of the state should be stored in a single location. This makes it easier to reason about your state and to track down bugs.
Time travel debugging
State management libraries often provide time-travel debugging capabilities. This allows you to step through the state of your application over time and to see how it changed. This can be a valuable tool for debugging problems in your application.
Best practices of state management in React Native
Here are some of the best practices of state management in React Native state management libraries:
1. Use immutable state:
As mentioned earlier, the state of your application should be immutable. This means that once you have set the state, you should not be able to change it directly. Instead, you should create a new state object with the changes you want to make. This makes it easier to reason about your state and to track down bugs.
2. Use selectors:
The UI of your application should be connected to the state through a series of functions called selectors. Selectors are functions that take the state as input and return a value. This makes it easier to test your UI and to make changes to the state without affecting the UI.
3. Avoid mutations:
Mutations are changes to the state that are made directly. Mutations are generally considered to be bad practices in state management because they can make it difficult to reason about the state and to track down bugs. Instead, you should use selectors to update the state in a controlled way.
4. Use middleware:
Middleware is a way to add additional functionality to state management libraries. Middleware can be used to do things like logging, validation, and caching.
5. Test your state management code:
It is important to test your state management code to ensure that it is working correctly. This will help you to avoid bugs and regressions in your application.
Most common mistakes when using state management libraries in React Native
Here are a few of the most common mistakes:
1. Not using a state management library at all:
If your application is small and simple, you may not need to use a state management library. However, if your application is complex or has a lot of state, you will need to use a state management library to keep your code organized and easy to maintain.
2. Using the wrong state management library:
There are a number of different state management libraries available for React Native. Each library has its own strengths and weaknesses, so it is important to choose the right library for your specific needs.
3. Not understanding the principles of state management:
State management libraries are based on a number of principles, such as immutability, encapsulation, and decoupling. If you do not understand these principles, you will not be able to use a state management library effectively.
4. Not following the best practices for state management:
There are a number of best practices for state management, such as using selectors, avoiding mutations, and using middleware. If you do not follow these best practices, your application will be more difficult to understand and maintain.
5. Not testing your state management code:
It is important to test your state management code to ensure that it is working correctly. This will help you to avoid bugs and regressions in your application.
Conclusion
Here are some general guidelines for choosing the right state management tool for your React Native app:
Small to medium-sized apps:
If you are building a small to medium-sized app, you can use a lightweight state management library like Zustand or Easy-peasy. These libraries are easy to learn and use, and they are well-suited for small to medium-sized applications.
Large applications:
If you are building a large application, you may need to use a more powerful state management library like Redux or Akita. These libraries provide a number of features that are not available in other libraries, such as code-splitting and hot reloading.
Simple applications:
If you are building a simple application with a limited number of state changes, you may not need to use a state management library at all. You can use the built-in React Context API to manage your state.
Complex applications:
If you are building a complex application with a large number of state changes, you will need to use a state management library. A state management library will help you to keep your state organized and make your application easier to debug.